Authenticating
How to authenticate with Orderful's API
Orderful's API requires that your API token is present in the headers of every request to our API. You do not need to generate the token, as it is automatically generated for you once you have created an account, and it can be located in the UI.
Step 1: Access your API Key from the UI
- Log in to https://ui.orderful.com/signup
- Navigate to Settings
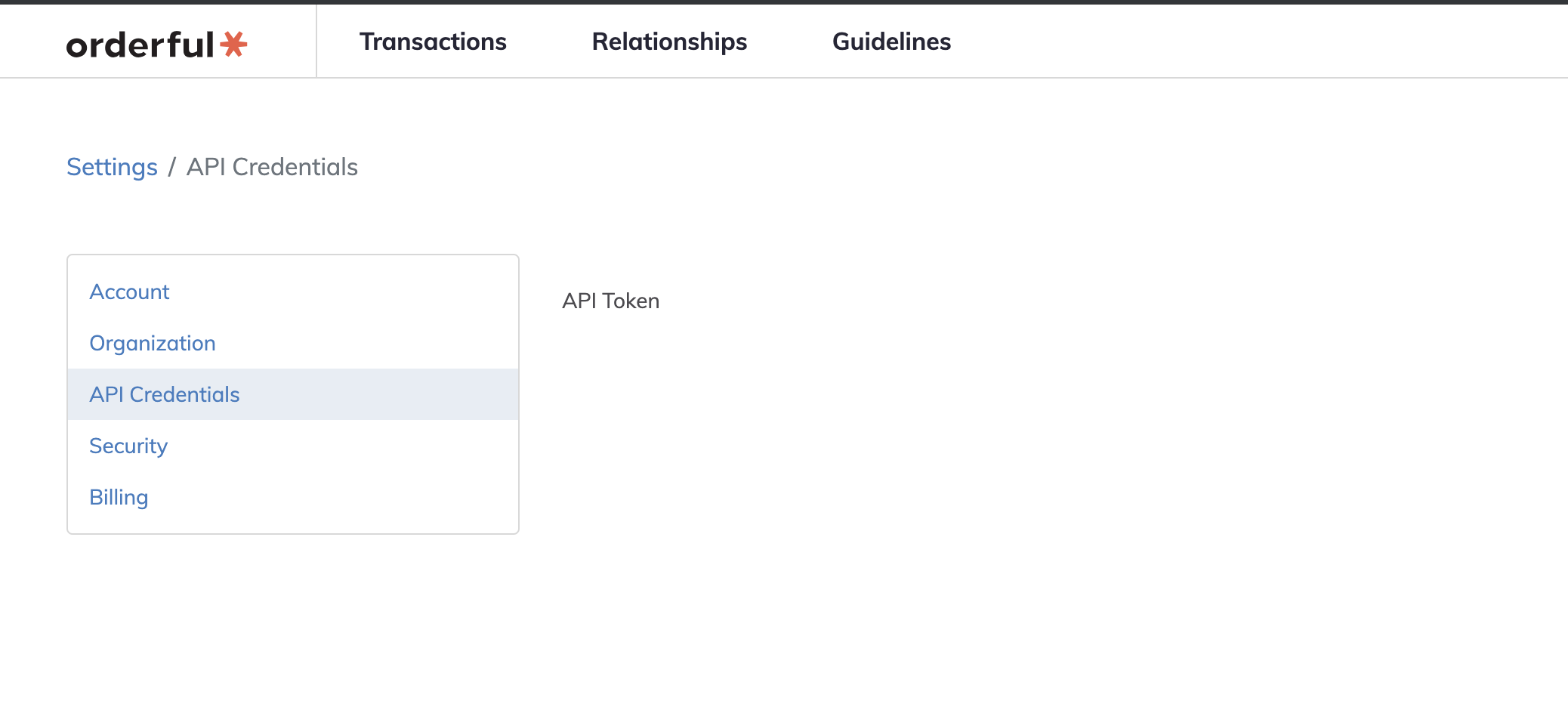
- Select API Credentials and Copy the Token Value
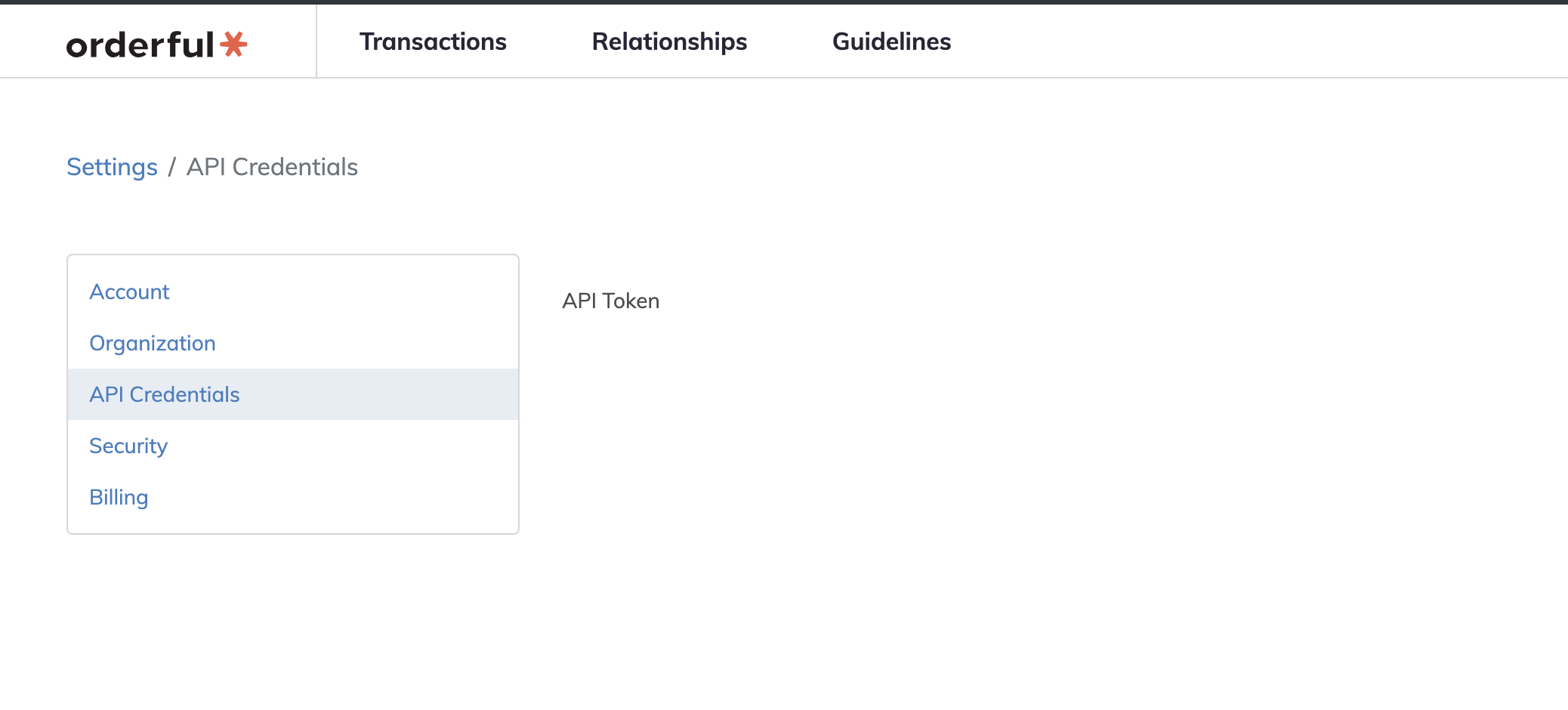
Step 2: Add the Token to the headers of your HTTP Requests
The expected header key is orderful-api-key. This key and the value (your API token that you copied from the UI) will need to be present in the headers of every HTTP request you make to the API.
Depending on what tool or method you are using to make HTTP requests, this will look different, but the net result is the same.
Your headers should include two keys:
orderful-api-key: api_token_value_from_ui_here
Content-Type: application/json
You will include the Content-Type header in the event that you are POSTing JSON to the API.
curl -H 'orderful-api-key: this_key_comes_from_the_UI' -H 'Content-Type': 'application/json'
headers = {"orderful-api-key": this_key_comes_from_the_UI, "Content-Type": "application/json"}
Postman example

Step 3: Make a Request
curl -X GET https://api.orderful.com/v2/transactions
import requests
requests.get('http://api.orderful.com/v2/transactions',headers=headers).text
'{"query":{"revision":"latest","ownerId":954,"offset":0,"limit":100},"pagination":{"offset":0,"limit":100,"total":0,"links":{"next":null,"prev":null}},"data":[]}'
Postman example
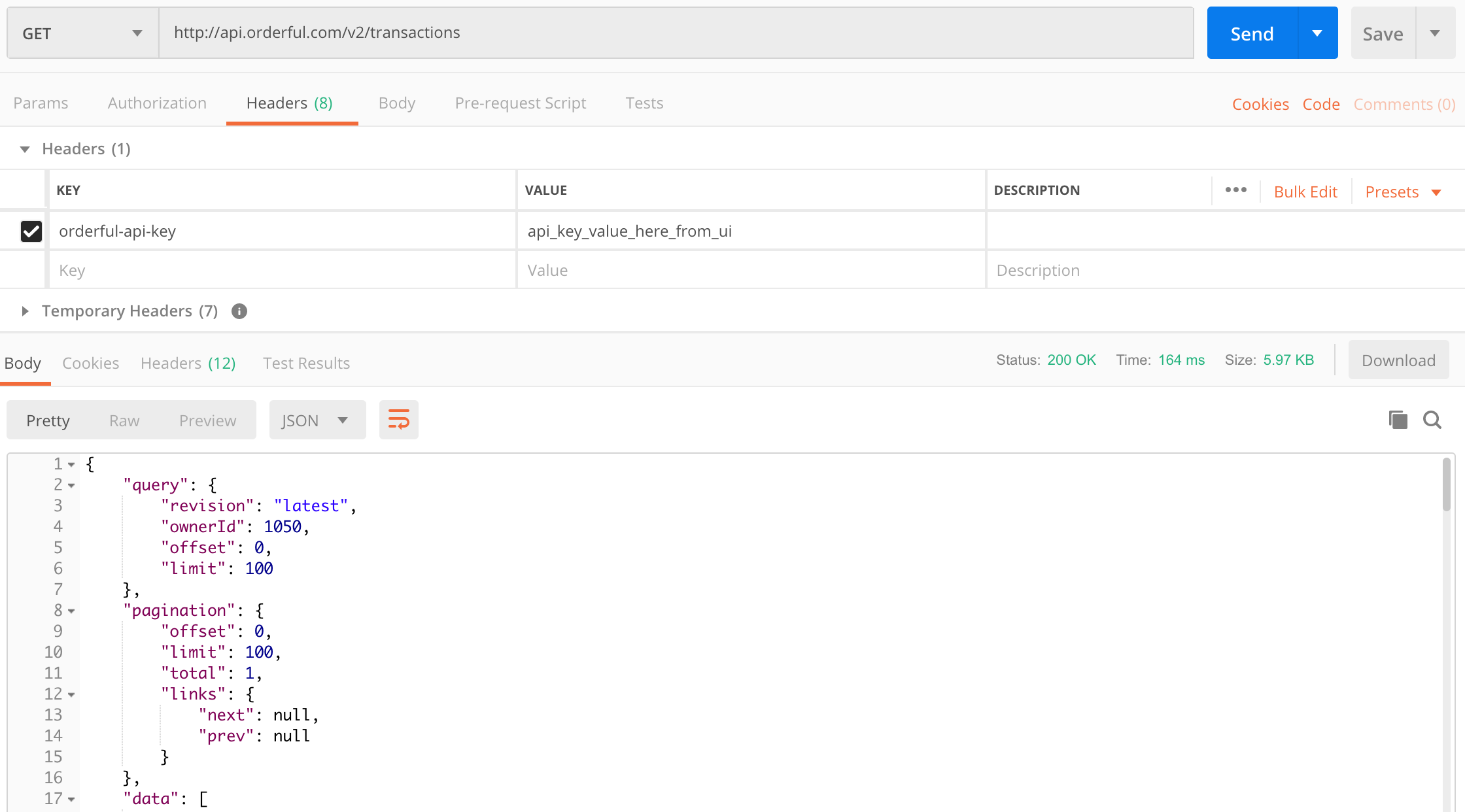
Full Workflow Example
curl -H 'orderful-api-key: this_key_comes_from_the_UI' -H 'Content-Type': 'application/json' -X GET https://api.orderful.com/v2/transactions
# the API key is from the UI after you've created an account.
api_key = 'this_key_comes_from_the_UI'
# set the required headers. orderful-api-key is required.
headers = {"orderful-api-key": api_key, "Content-Type": "application/json"}
# Example: send a request to get all current transactions
In [114]: requests.get('http://api.orderful.com/v2/transactions',headers=headers).text
Out[114]: '{"query":{"revision":"latest","ownerId":954,"offset":0,"limit":100},"pagination":{"offset":0,"limit":100,"total":0,"links":{"next":null,"prev":null}},"data":[]}'
Updated almost 5 years ago